위로
아래
효과 메소드
// 숨김
hide() // 요소 숨기기
fadeOut() // 요소가 점점 투명해지면서 사라진다
slideUp() // 요소가 위로 접히며 숨겨진다
// 노출
show() // 숨겨진 요소가 노출
fadeln() // 숨겨진 요소가 점점 선명
slideDown() // 숨겨진 요소가 아래로 펼쳐짐
// 노출, 숨김
toggle() // hide, show 효과 적용
fadeToggle() // fadeIn, fadeOut 효과 적용
slideToggle() // slideUp, sLideDown 효과 적용
fadeTo() // 지정한 투명도 적용
//속성
$("p").slideUp(1000,linear,fuction(){})
1000 : 효과 소요 시간 1초 (slow, normal, fast)
linear : 일정한 속도 (swing, linear)
function(){} : 콜백함수
예시
더보기

<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="../js/jquery-3.7.0.min.js"></script>
<script type="text/javascript">
$(function(){
$(".btn2").hide();
$(".btn1").on("click", function(){
$(".box").slideUp(1000,"linear", function(){
$(".btn1").hide();
$(".btn2").show();
});
});
$(".btn2").on("click", function(){
$(".box").fadeIn(1000,"swing",function(){
$(".btn2").hide();
$(".btn1").show();
});
});
$(".btn3").on("click",function(){
$(".box").slideToggle(1000,"linear");
});
$(".btn4").on("click",function(){
$(".box").fadeTo("fast", 0.3);
});
$(".btn5").on("click", function(){
$(".box").fadeTo("fast",1);
});
})
</script>
<style>
.content{width:400px; background-color: #eee;}
</style>
</head>
<body>
<p>
<button class="btn1">slideUp</button>
<button class="btn2">fadeIn</button>
</p>
<p>
<button class="btn3">toggleSlide</button>
</p>
<p>
<button class="btn4">fadeTo(0.3)</button>
<button class="btn5">fadeTo(1)</button>
</p>
<div class="box">
<div class="content">
우리는 두 수의 최대값을 구하는 경우에 Math클래스를 사용하는데, static 메소드로 선언된 max 함수를 초기화 없이 사용합니다. 하지만 static 메소드에서는 static이 선언되지 않은 변수에 접근이 불가능한데, 메모리 할당과 연관지어 생각해보면 당연합니다. 우리가 Test.printName() 을 사용하려고 하는데, name1은 new 연산을 통해 객체가 생성된 후에 메모리가 할당됩니다. 하지만 static 메소드는 객체의 생성 없이 접근하는 함수이므로, 할당되지 않은 메모리 영역에 접근을 하므로 문제가 발생하게 됩니다. 그러므로 static 메소드에서 접근하기 위한 변수는 반드시 static 변수로 선언되어야 합니다.
</div>
</div>
</body>
</html>

animate 메소드
기본형
$("요소 선택").animate({스타일 속성}, 적용 시간, 가속도, 콜백 함수)
$("div").animate({marginLeft : "100px"}, 1000, "linear", function(){alert("끝")})
position 위치를 바꿔주려면, css의 기본 설정 position이 static으로 설정되어 있는 걸 바꿔야 한다.
예시
더보기
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="../js/jquery-3.7.0.min.js"></script>
<script type="text/javascript">
$(function(){
$(".btn1").on("click",function(){
$(".txt1").animate({
marginLeft: "500px",
fontSize: "30px"
},2000,"linear",function(){
alert("모션 완료!");
});
});
$(".btn2").on("click", function(){
$(".txt2").animate({
marginLeft:"+=50px"
}, 1000);
});
});
</script>
</head>
<body>
<p><button class="btn1">버튼1</button></p>
<span class="txt1">"500px" 이동</span>
<p><button class="btn2">버튼2</button></p>
<span class="txt2">"50px" 이동</span>
</body>
</html>
delay, stop, stop(true,true) 예시
더보기
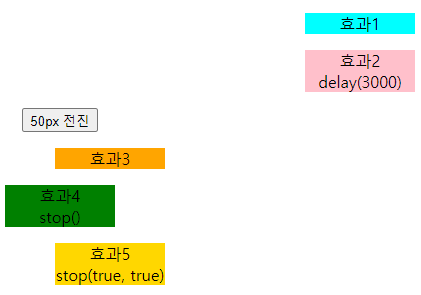
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="../js/jquery-3.7.0.min.js"></script>
<style>
p {width:110px;text-align:center;}
.txt1 {background-color: aqua;}
.txt2 {background-color: pink;}
.txt3 {background-color: orange;}
.txt4 {background-color: green;}
.txt5 {background-color: gold;}
</style>
<script type="text/javascript">
$(document).ready(function(){
$(".txt1").animate({marginLeft:"300px"},1000);
$(".txt2").delay(3000).animate({marginLeft:"300px"},1000);
$(".btn1").on("click",moveFnc);
function moveFnc() {
$(".txt3").animate({marginLeft: "+=50px"},800);
$(".txt4").animate({marginLeft: "+=50px"},800);
$(".txt4").stop();
$(".txt5").animate({marginLeft :"+=50px"},800);
$(".txt5").stop(true, true);
}
})
</script>
</head>
<body>
<p class="txt1">효과1</p>
<p class="txt2">효과2<br>delay(3000)</p>
<p><button class="btn1">50px 전진</button></p>
<p class="txt3">효과3</p>
<p class="txt4">효과4<br>stop()</p>
<p class="txt5">효과5<br>stop(true, true)</p>
</body>
</html>
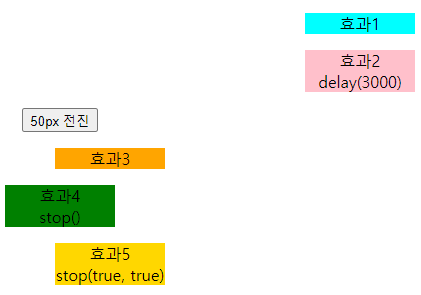
queue, dequeue 예시
더보기
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="../js/jquery-3.7.0.min.js"></script>
<style type="text/css">
* {margin:0;}
.txt1 {width:50px; text-align:center;background-color:aqua;}
</style>
<script type="text/javascript">
$(function(){
$(".txt1").animate({marginLeft:"200px"},1000)
.animate({marginTop:"200px"},1000)
.queue(function(){
$(this).css({"background":"red"});
$(this).dequeue();
}).animate({marginLeft:"0"},1000).animate({marginTop:"0px"},1000);
})
</script>
</head>
<body>
<p class="txt1">내용1</p>
</body>
</html>
clearQueue 예시
더보기
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="../js/jquery-3.7.0.min.js"></script>
<style type="text/css">
.txt1, .txt2 {width:50px; text-align:center; background-color:aqua;}
.txt2 {background-color:orange;}
</style>
<script type="text/javascript">
$(document).ready(function(){
$(".txt1").animate({marginLeft:"100px"},1000)
.animate({marginLeft:"300px"},1000)
.animate({marginLeft:"400px"},1000)
$(".txt2").animate({marginLeft:"100px"},1000)
.animate({marginLeft:"300px"},1000)
.animate({marginLeft:"400px"},1000)
$(".txt2").clearQueue();
})
</script>
</head>
<body>
<p class="txt1">내용1</p>
<p class="txt2">내용2</p>
</body>
</html>